Python OCR SDK Package Dev Guide¶
Asprise OCR for Python Installation¶
There are two options: install using pip or manually download the SDK.
Option 1: Install with pip¶
pip is a popular Python package installer. To install Asprise OCR SDK with pip, simply execute:
sudo pip install asprise_ocr_sdk_python_api # Linux and Mac
For Microsoft Windows users, please use:
pip install asprise_ocr_sdk_python_api
A demo front-end GUI for the OCR engine is installed as a script named asprise_ocr
.
You can run the following command in the same shell/console:
asprise_ocr
Note although the demo program has a dependency on TkInter, the core Python OCR API doesn’t.
Once the demo UI launches, you can perform OCR easily by selecting an image file and hitting the OCR button:
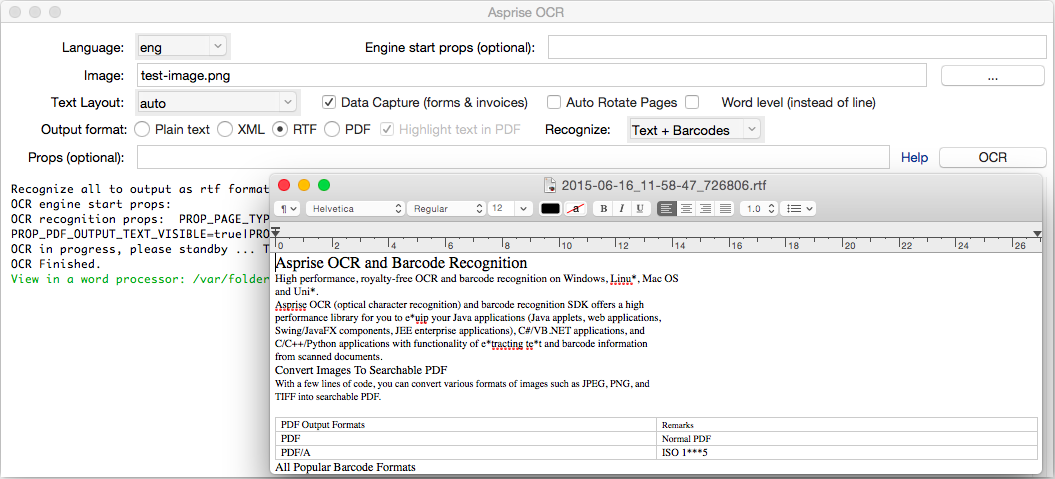
After the UI lauches, you might be asked to perform a one time data library download.
Later sections will introduce how to invoke the OCR API without the UI.
Option 2: Python OCR package direct download¶
Download a copy of Asprise OCR SDK from http://www.asprise.com/product/ocr. Unzip it and that’s all.
After unzipping the package, run the commands to launch the OCR demo program.
cd asprise_ocr_api
python ocr_app.py
Jump Start¶
The following code demonstrates the basic usage of Python OCR:
1 2 3 4 5 6 7 8 9 10 | from asprise_ocr_api import *
Ocr.set_up() # one time setup
ocrEngine = Ocr()
ocrEngine.start_engine("eng")
s = ocrEngine.recognize("test-image.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_ALL, OCR_OUTPUT_FORMAT_PLAINTEXT)
print "Result: " + s
# recognizes more images here ..
ocrEngine.stop_engine()
|
Line 1: Imports the Ocr class and constants.
Line 4 and 5: Creates a new Ocr engine and starts it for recognizing English. The evaluation version is able to recognize English (eng), Spanish (spa), Portuguese (por), German (deu) and French (fra). For other languages, please contact us. The list of languages supported can be found Languages Supported.
Line 6: All the OCR work is done here. The recognizeAll method of the com.asprise.util.ocr.OCR class recognizes all the characters and barcodes
from the image and output them as a string. The output format is set as plain text. Other supported formats are: XML (Ocr.OUTPUT_FORMAT_XML
),
searchable PDF (Ocr.OUTPUT_FORMAT_PDF
) and user editable RTF (Ocr.OUTPUT_FORMAT_RTF
).
Input and Output¶
Asprise OCR supports the following image formats: GIF, PNG, JPEG, TIFF and PDF.
For the sample OCR code in above section, if the input looks like below:
[Download the actual input image here]
The OCR output will be:
Plain-text Format¶
Asprise OCR and Barcode Recognition
High performance, royalty-free OCR and barcode recognition on Windows,
...
ISBN-l3, Interleaved 2 of 5, Code 39, Code 128, PDF417, and QR Code.
[[QR-Code: www.asprise.com]]
[[CODE-128: Asprise]].
The last two lines represents bar code information extract. Note both the format and content of the barcode are enclosed in ‘[[ ]]’ pairs.
XML Format¶
Asprise OCR XML output contains rich information like text coordinates, fonts, confidence level, barcode type, barcode locations, as well as table information (cells with location and row/column info).
Set the output format to OUTPUT_FORMAT_XML, and the you’ll get:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | <?xml version="1.0"?>
<?xml-stylesheet type="text/xsl" href="aocr.xsl"?>
<asprise-ocr input="test-image.png">
<page no="0" width="2400" height="3200" words="126" confidence="92" time-taken="1.928">
<block id="1e" type="text" subtype="line" x="320" y="248" width="1176" height="63" words="5" confidence="93" font-name="Times_New_Roman" font-size="66" font-bold="0" font-italic="0" font-serif="1" baseline="297" xheight="34" ascent="15" descent="-15">Asprise OCR and Barcode Recognition</block>
<block id="1f" type="text" subtype="line" x="320" y="360" width="1725" height="43" words="13" confidence="92" font-name="Times_New_Roman" font-size="45" font-bold="0" font-italic="0" font-serif="1" baseline="394" xheight="23" ascent="11" descent="-10">High performance, royalty-free OCR and barcode recognition on Windows, Linux, Mac OS</block>
<!-- ... -->
<table id="4" x="320" y="1083" width="1766" height="169" rows="3" cols="2" cells="6" words="10" confidence="91">
<cell id="3" x="320" y="1083" width="563" height="57" row="0" col="0" rowspan="1" colspan="1">
<block id="29" type="text" subtype="line" x="343" y="1098" width="429" height="41" words="3" confidence="92" font-name="Times_New_Roman" font-size="41" font-bold="0" font-italic="0" font-serif="1" baseline="1130" xheight="23" ascent="9" descent="-8">PDF Output Formats</block>
</cell>
<cell id="5" x="883" y="1083" width="1203" height="57" row="0" col="1" rowspan="1" colspan="1">
<block id="2a" type="text" subtype="line" x="905" y="1099" width="181" height="32" words="1" confidence="90" font-name="Times_New_Roman" font-size="37" font-bold="0" font-italic="0" font-serif="1" baseline="1130" xheight="22" ascent="9" descent="-5">Remarks</block>
</cell>
<cell id="6" x="320" y="1140" width="563" height="55" row="1" col="0" rowspan="1" colspan="1">
<block id="2b" type="text" subtype="line" x="343" y="1154" width="83" height="31" words="1" confidence="93" font-name="Times_New_Roman" font-size="43" font-bold="0" font-italic="0" font-serif="1" baseline="1185" xheight="21" ascent="10" descent="-10">PDF</block>
</cell>
<!-- ... -->
<block id="1" type="barcode" subtype="QR-Code" x="1397" y="1647" width="441" height="441" confidence="1">www.asprise.com</block>
<block id="2" type="barcode" subtype="CODE-128" x="348" y="1651" width="583" height="147" confidence="148">Asprise</block>
</page>
</asprise-ocr>
|
You may view the result in a browser. To do so, you need to save the XML into a file (e.g. ocr-result.xml) and download http://asprise.com/ocr/files/schema/15/aocr.xsl to the same folder as the XML file. Then open the XML file using IE, Firefox or Safari (Chrome doesn’t support this):
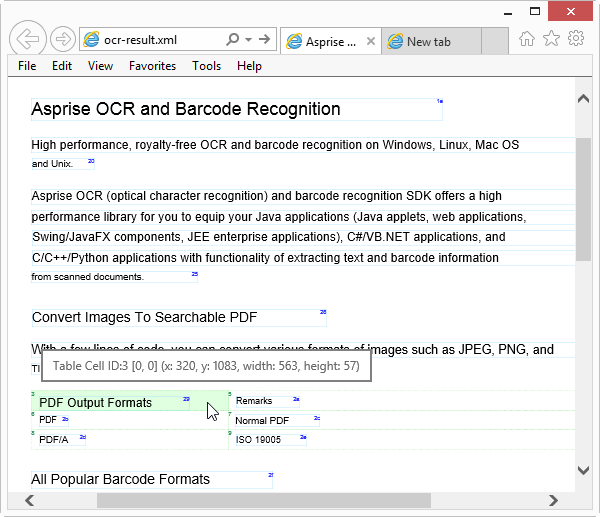
Searchable PDF Format¶
If you set the output format as OUTPUT_FORMAT_PDF, you need to specify the target PDF output file as following:
1 2 3 4 | ocrEngine.recognize("test-image.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_ALL, OCR_OUTPUT_FORMAT_PDF,
PROP_PDF_OUTPUT_FILE="ocr-result.pdf",
PROP_PDF_OUTPUT_TEXT_VISIBLE=True)
|
In above code, properties are specified using keyword arguments.
For the list of all supported properties, please refer to Asprise OCR Property Summary.
Once the OCR done, you can open the PDF output file with any PDF viewer and perform searches:
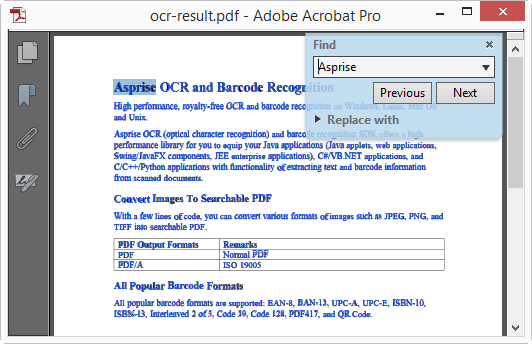
To make the text invisible or transparent, you simply set PROP_PDF_OUTPUT_TEXT_VISIBLE
to False.
Both normal PDF and PDF/A are supported. Please refer to the options guide.
Rich Text Format (RTF)¶
Set the output format as OUTPUT_FORMAT_RTF, you can then output .rtf files that can be edited in most word processors (Microsoft Word, Libre Office, TextEdit, etc.).
1 2 3 | ocrEngine.recognize("test-image.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_ALL, OUTPUT_FORMAT_RTF,
PROP_RTF_OUTPUT_FILE="ocr-result.pdf")
|
Once the OCR done, you can open the RTF file with a word processor:
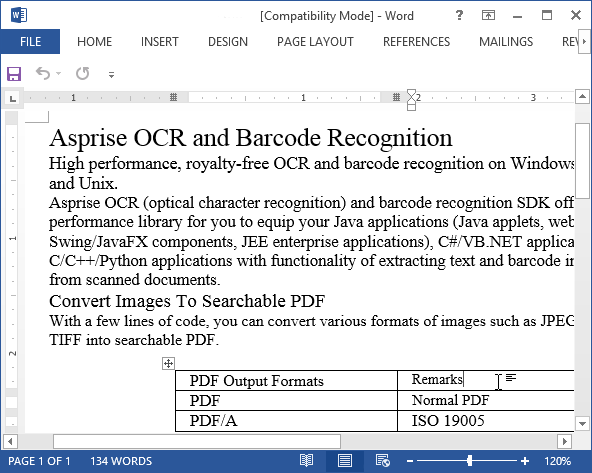
OCR In Action¶
This section covers common OCR tasks.
Recognizes text only or barcode only¶
To save OCR time, you can choose to OCR text or barcode only:
Text only:
s = ocrEngine.recognize("test.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_TEXT, OCR_OUTPUT_FORMAT_PLAINTEXT)
Barcode only:
s = ocrEngine.recognize("test.png", -1, -1, -1, -1, -1,
RECOGNIZE_TYPE_BARCODE, OCR_OUTPUT_FORMAT_PLAINTEXT)
Perform OCR on part of the image¶
In some cases, you might not want to OCR the whole image. In that case, you can OCR on part of the image to save time:
s = ocrEngine.recognize("test.png", -1, 0, 0, 400, 200,
OCR_RECOGNIZE_TYPE_TEXT, OCR_OUTPUT_FORMAT_PLAINTEXT)
The above code OCR the top left part of the image with width 400 pixels and height 200 pixels.
Perform OCR on multiple input files in one shot¶
You can perform OCR on multiple files in one shot:
s = ocrEngine.recognize("test1.png;test2.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_TEXT, OCR_OUTPUT_FORMAT_PLAINTEXT)
Perform OCR on a certain page from the specified TIFF file¶
A TIFF file may contain multiple pages. If you need to recognize only a certain page, you can specify the page number as following:
s = ocrEngine.recognize("test.tif", 1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_TEXT, OCR_OUTPUT_FORMAT_PLAINTEXT)
Note 1
means the second page (the page number of the first page is 0). -1
means all pages.
Perform OCR on a PDF input file¶
You use the following method to perform OCR on a PDF input file:
s = ocrEngine.recognize("test.pdf", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_TEXT, OCR_OUTPUT_FORMAT_PLAINTEXT)
Improve OCR Accuracy¶
Scan in Grayscale with DPI 300¶
If the input images are from scanners, please follow the guidelines below:
Scan at resolution DPI 300 (or 400 for small fonts). Higher DPI may not necessarily result in better accuracy but lower DPI may affect the quality.
Scan in grayscale mode or color mode, but not black/white mode.
Pre-Process Images¶
Image pre-processing can be a great tool to improve OCR accuracy for special images.
Images with very small font¶
Enlarge it:
ocrEngine.recognize("test-image.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_ALL, OCR_OUTPUT_FORMAT_XML,
PROP_IMG_PREPROCESS_TYPE="custom",
PROP_IMG_PREPROCESS_CUSTOM_CMDS="scale(2);default()") # scale up
Images with light text on a dark background¶
Invert it:
ocrEngine.recognize("test-image.png", -1, -1, -1, -1, -1,
OCR_RECOGNIZE_TYPE_ALL, OCR_OUTPUT_FORMAT_XML,
PROP_IMG_PREPROCESS_TYPE="custom",
PROP_IMG_PREPROCESS_CUSTOM_CMDS="invert();default()") # invert color
Image operations specified PROP_IMG_PREPROCESS_CUSTOM_CMDS
are executed in a
chain. You can chain
many opertions in the command. The list of available operations can be found at Image Pre-processing Related Properties.
Provide Dictionaries or Templates¶
The OCR engine is optimized to recognize human readable words and there are built-in dictionaries and templates. We’ll use an example to find out roles played by dictionaries.
For example, the image below contains two words: abcdopqr abcd0pqr
:
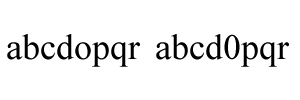
The OCR result with the default settings will be: abcdopqr abchpqr
. The OCR engine may have detected abcd0pqr
but it discarded it
as it is unlikely a word since there is a number between characters.
Suppose we do consider abcd0pqr
as a word. In such case, we can provide our own dictionary file:
abcdopqr
abcd0pqr
Note word entries are separated by line breaks. Save the above content into a file dict.txt
and specify it when you start the OCR engine:
ocrEngine = Ocr()
ocrEngine.start_engine("eng",
START_PROP_DICT_CUSTOM_DICT_FILE="dict.txt")
ocrEngine.recognize# ...
Note this property must be specified when you call start_engine
. Now, you’ll get the extact text as on the image: abcdopqr abcd0pqr
Alternatively, you can specify the words using a template:
abcd\\npqr
Template entries are separated by line breaks. Save it to a file templates.txt
and specify it using :
ocrEngine = Ocr()
ocrEngine.start_engine("eng",
START_PROP_DICT_CUSTOM_TEMPLATES_FILE="templates.txt")
ocrEngine.recognize# ...
Again, the correct result is returned.
The following wildcards are allowed in templates:
|
Any alphbet character |
|
Digit (0-9) |
|
Alphabet or digit |
Multi-threading with Asprise OCR¶
Most of modern computers run on multi-core CPUs. Multi-threading can significantly reduce the runtime by making full use of the processor power.
You may take advantage of Python’s threading package. Note that each thread must have its own dedicated OCR engine instance.
Software Packaging and Distribution¶
So you have successfully developed your Python applications with Asprise OCR. It’s time to distribute your programs to end users. First, make sure you are an authorized licensee registered with Asprise. To purchase a license, please visit: http://www.asprise.com/product/ocr